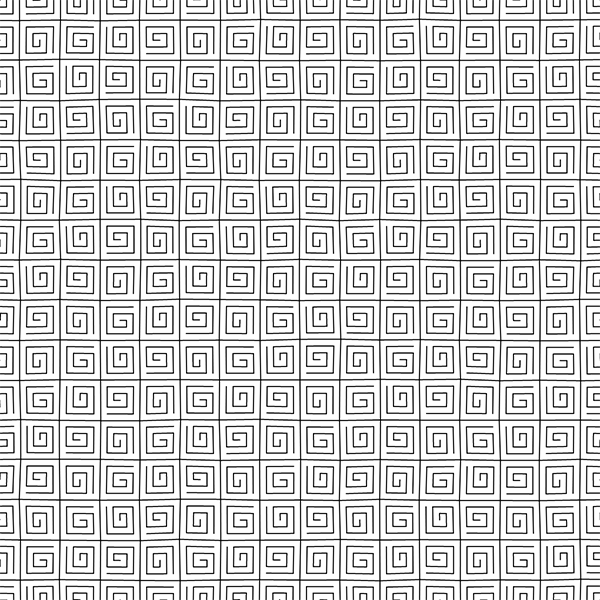
The Entanglement library now supports Ambler! This officially doubles the number of tangles it can produce! Two tangles! Progress! OK, so two tangles isn’t really all that many, but still! Progress!
Using Entanglement to draw a basic Ambler is easy. Here’s the program that generated the image at the top of this page:
const height = 600;
const width = 600;
function setup() {
createCanvas(width, height);
background(255);
}
function draw() {
let amb = new Ambler(width, height, {});
amb.paste(new Point(0, 0));
noLoop();
}
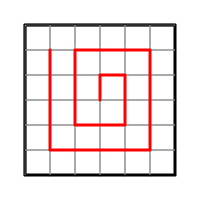
That’s a lot of calculation to go through – it seems like there should be a less computationally-intensive way to draw those lines. There is, if you know the square is actually square! But we’re going to warp our square somewhat, and this way the spiral can be drawn no matter what four points are used as corners. We’ll see an example of that shortly.
So drawing Ambler is as simple as creating the grid and drawing a box spiral in each section. In subsequent sections, we rotate the spiral 90 degrees.
To make the result look more hand-drawn, there is some random slop built into where the points on the grid are. It’s very slight by default, but we could make it much larger for some interesting effects. There is a gridSpacing option that specifies how far apart the grid lines are. By default, this is set to 40. Then there is a gridVary option which sets how many pixels each grid point could move left, right, up or down. If you set gridVary to one half of gridSpacing, you get an interesting effect:
const amb = new Ambler(width, height, {
gridVary: 20,
});
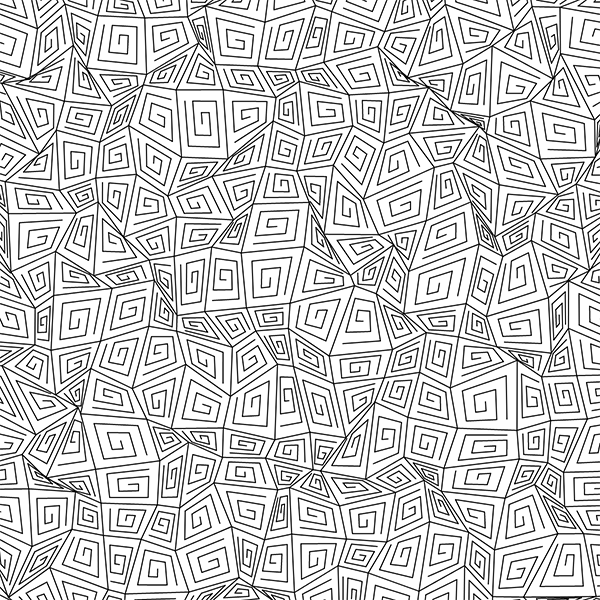
Increasing gridVary to more than half of gridSpacing causes some overwriting of adjacent sections as some of the “squares” can get twisted inside out. Here, gridVary is more than gridSpacing:
const amb = new Ambler(width, height, {
gridVary: 50,
});
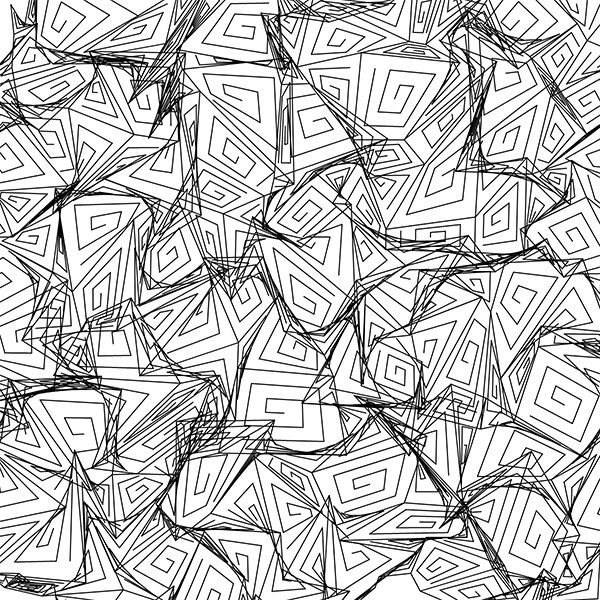
Breaking Changes
Adding a second tangle to the library pointed out many opportunities for consolidating and refactoring the code, and highlighted some poor choices I made early on. As a result, there have been many changes to how the library works since the first post about Entanglement. In particular, none of the examples there work anymore! The major change from the user’s point of view is that we no longer specify a box on the canvas to draw a tangle into. Instead, the user sets the size of the area, and “draws” the tangle into a graphics buffer. Later this buffer can be pasted onto the canvas at any place, potential at several places if needed. The examples in the documentation have been updated to show the new process.
If you want to use the old examples from the first post, specify version 0.0.1 in your HTML file:
<script src="https://cdn.jsdelivr.net/gh/tektsu/entanglement@0.0.1/dist/entanglement.js"></script>
For a version that works with the examples in this post, use:
<script src="https://cdn.jsdelivr.net/gh/tektsu/entanglement@0.0.2/dist/entanglement.js"></script>
In future posts about Entanglement, I’ll post the correct line to use at the top of the post.
This will probably keep happening for a while, as we are very early in the lifecycle of this library! If old examples don’t work, check the latest documentation! As we progress, this will happen less and less.