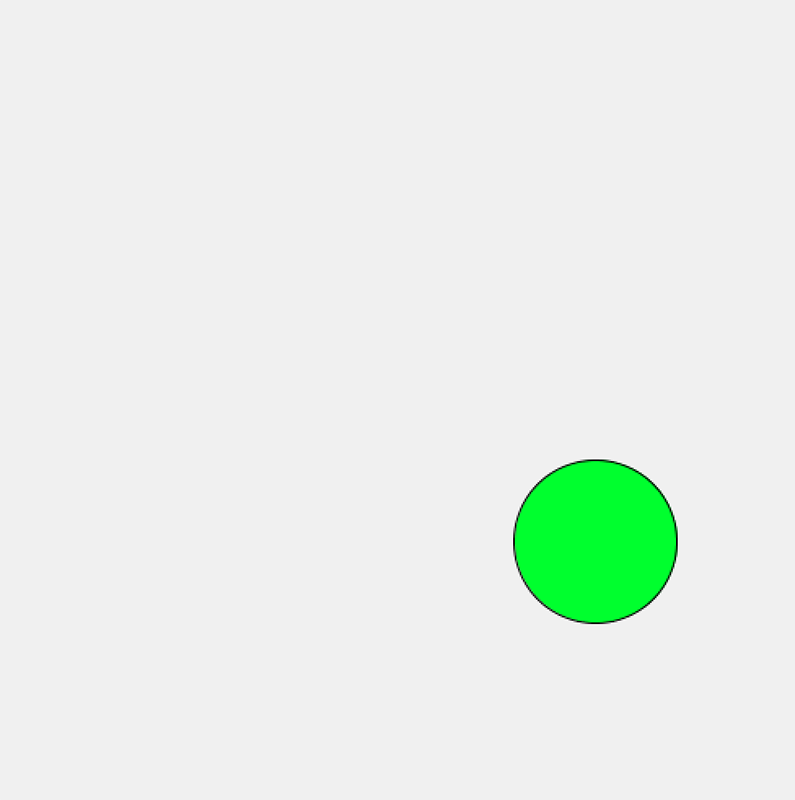
If you’ve been reading my posts each week, you’re probably aware that I have been working mostly in Javascript, using the p5.js drawing library. There are many things to like about Javascript, but also many ways for it to slowly drive you bonkers. I won’t go into all those reasons here, but I will say it’s time to try something new. I’d like to try to do some generative art using the Clojure language. This is way out of my comfort zone, since I don’t actually know Clojure, and it is not a simple language, but I thought I’d share my experiences with it here.
What is Clojure?
Clojure is a functional programming language, and as such is declarative. This differs quite a bit from most programming languages, like Basic, C, C++, Java, Python, etc, which are imperative or procedural. In an imperative language, you write a program that implements an algorithm – you tell the the computer how to solve a problem or complete a task. i.e. First do this, then do this, etc. In a functional or declarative language, computation is treated as the evaluation of mathematical functions. Functions are self-contained and do not share state, meaning data is not shared between functions. This means that to understand a function, all you need to look at is that function, it doesn’t depend on other parts of the program. Fun fact: Clojure has variables, but they never vary! Variables are immutable – once you set them, they never change (so are they really variables?) This differs from most imperative languages that keep track of program state by modifying variables.
Variables that don’t vary? “How does that work?”, you ask. Well, I’m not going to tell you! My goal here is to explore some generative art techniques using Clojure, not try to teach Clojure. If you’d like to learn more about Clojure, check out Closure For The Brave And The True , and excellent introduction to the language and functional programming. The book can be ordered from Amazon , or is available to read online for free.
Clojure actually runs on the Java Virtual Machine, which means that to use Clojure, you have to have Java installed (most computers already do!) This allows Closure to have access to pretty much all the resources that Java has, and that is extensive. More on that later…
By the way, those of you who are old (like me) and have been programming for decades (like me) may look at Clojure and say “Hey! That’s LISP!” You’re not wrong! Clojure is a dialect of that venerable 1950’s-era language: Lisp, so if you have used Lisp (or Scheme) before, you won’t have any trouble with Clojure.
Quil
“But Javascript with p5.js was cool! p5.js has all kinds of nifty drawing features! What does Clojure have that is as nice? Are you leading us astray???” Oh, quit whining. The p5.js library is part of the Processing project. Clojure has the Quil library, which is based on Processing! So many of the p5.js features will be found in Quil, and they will work very similarly.
Getting Started
We’re going to do a basic set up Clojure and Quil, and run a quick demomstration.
First you need Java! You probably already have it. I am using Java 8 (also known as Java 1.8, for some reason.) Any Java should work, but I found I had some problems using the OpenJDK 14.0 version of Java, so probably avoid that one…
Second, install Clojure (instructions for your specific kind of computer are here ).
Next, install Leiningen . This is a utility to help you build and run Clojure projects.
Now create your first project! Go to your command line and run:
lein new quil first-project
That command will create a directory called first-project
with a bunch of files and other directories in it. Change to that directory.
cd first-project
Run the REPL. “What’s a REPL?” REPL is “Read/Evaluate/Print/Loop”. This a program that lets you type Clojure expressions to be evaluations and displayed.
lein repl
You should see, well, a bunch of stuff, but then a prompt that looks like this:
user=>
At that prompt, type this cryptic incantation:
(use 'first-project.core :reload-all)
If all goes well, a window should open, displaying this wondrous work of art:
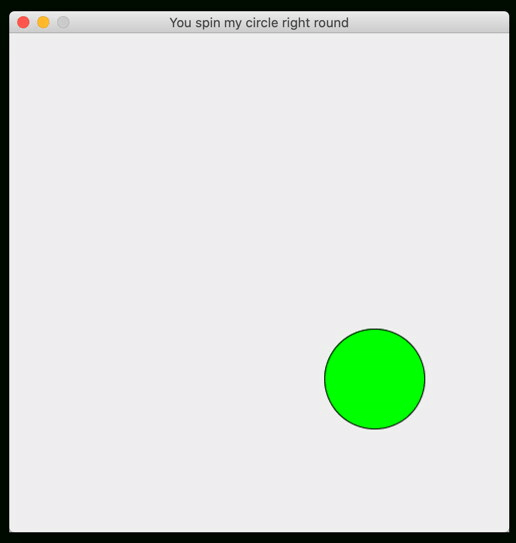
That’s all we’ll do for now! If you got that far, you have accomplished quite a bit! If you really want to look at the program, it’s in the file first-project/src/first_project/core.clj
, but you might want to start learning some Clojure first before jumping down that particular rabbit hole.
Next time we’ll try to do something a bit more interesting and “arty”.